Building Fast, Responsive Web Applications with Tailwind CSS and Svelte
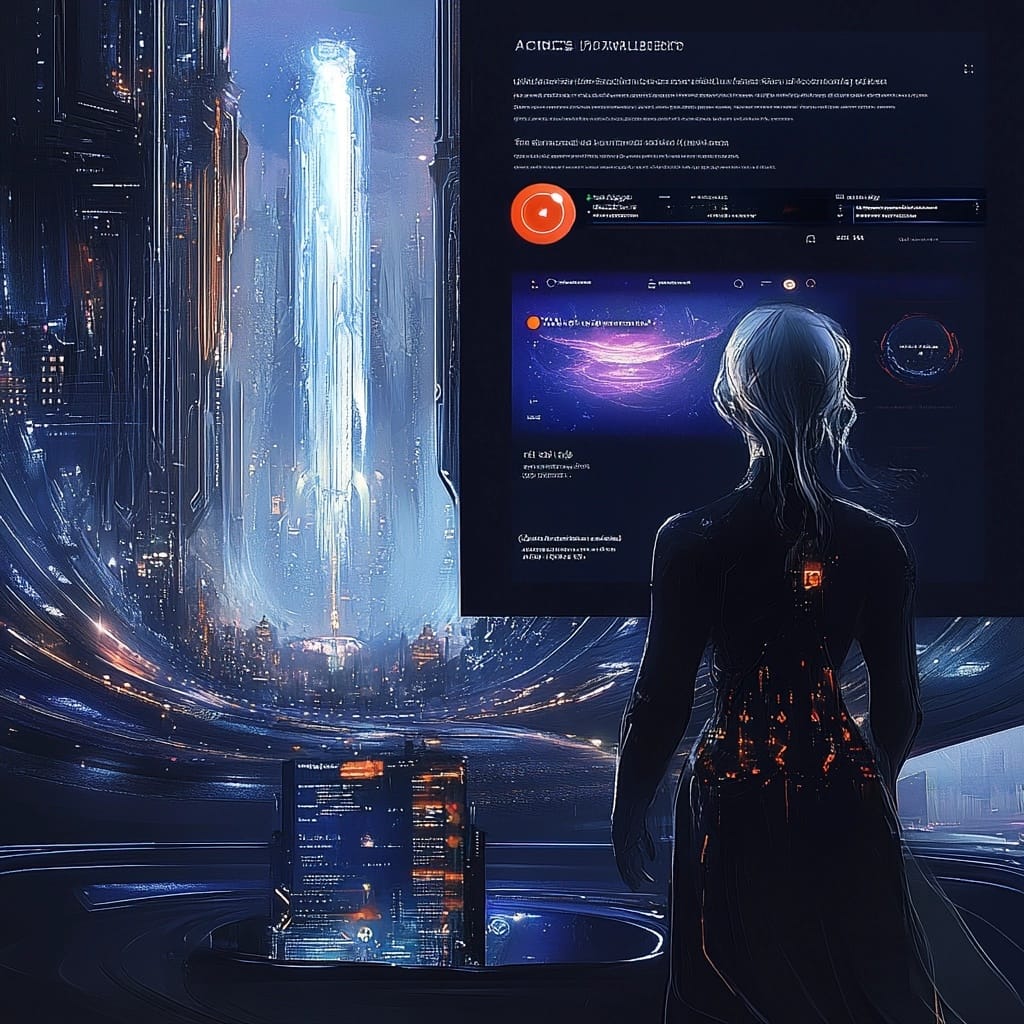
Writing maintainable CSS has long been a pain point in web development. Traditional approaches, while functional, often come with significant challenges:
- BEM Methodology: Leads to verbose class names like
button__icon--large
and repetitive typing. - SASS/SCSS Nesting: Can quickly spiral into complexity (e.g.,
nav > ul > li > a
). - CSS-in-JS: Adds runtime overhead and often requires additional tooling.
- Custom Naming Conventions: Hard to enforce consistency across teams ("Is it
primary-button
orbuttonPrimary
?"). - Global CSS Scope: Introduces constant battles with specificity.
- Component Frameworks: Often require learning proprietary styling systems.
Add to this the challenge of building performant applications, and the result is often a cumbersome workflow. Enter Tailwind CSS and Svelte, a modern duo designed to simplify development while delivering exceptional performance.
Why Tailwind CSS and Svelte Work So Well Together
This stack excels by addressing core developer pain points:
- Tailwind CSS: Eliminates CSS maintenance headaches with utility classes, enabling developers to focus on building rather than styling.
- Svelte: Optimizes DOM updates by compiling components ahead of time, resulting in faster applications.
- Combined Power: Together, they reduce development time and runtime overhead, making them a perfect pair for modern web development.
Setting Up Your Project
Getting started with SvelteKit and Tailwind CSS is straightforward using the SvelteKit CLI. Here’s how to set up your project:
# Create a new SvelteKit project
npx sv create my-app
# During the setup process:
# 1. Choose "SvelteKit minimal"
# 2. Select TypeScript if desired
# 3. Select "tailwindcss" from the additional tools checklist (space bar to select)
# Navigate to your project
cd my-app
# Install dependencies
npm install
# Start the development server
npm run dev
If you miss selecting Tailwind CSS during the setup, you can easily add it later:
# Install Tailwind and its dependencies
npm install -D tailwindcss postcss autoprefixer
# Initialize Tailwind
npx tailwindcss init -p
Update your tailwind.config.js
to specify where Tailwind should look for classes:
/** @type {import('tailwindcss').Config} */
export default {
content: ["./src/**/*.{html,js,svelte,ts}"],
theme: {
extend: {},
},
plugins: [],
}
Finally, include the Tailwind directives in your src/app.css
file:
@tailwind base;
@tailwind components;
@tailwind utilities;
Building a Real Component: Modal Example
Here’s how to build a practical modal component that incorporates responsive design, state management, and accessibility.
Modal.svelte:
<script>
export let isOpen = false;
export let title = '';
function close() {
isOpen = false;
}
function handleEscape(e) {
if (e.key === 'Escape') close();
}
</script>
<svelte:window on:keydown={handleEscape}/>
{#if isOpen}
<div
class="fixed inset-0 bg-black bg-opacity-50 flex items-center justify-center p-4"
on:click|self={close}
>
<div
class="bg-white rounded-lg max-w-md w-full p-6 shadow-xl"
role="dialog"
aria-modal="true"
aria-labelledby="modal-title"
>
<div class="flex justify-between items-center mb-4">
<h2 id="modal-title" class="text-xl font-semibold">{title}</h2>
<button
class="text-gray-500 hover:text-gray-700"
on:click={close}
aria-label="Close modal"
>
×
</button>
</div>
<div class="mt-4">
<slot />
</div>
<div class="mt-6 flex justify-end space-x-4">
<button
class="px-4 py-2 text-gray-600 hover:text-gray-800"
on:click={close}
>
Cancel
</button>
<button
class="px-4 py-2 bg-blue-500 text-white rounded hover:bg-blue-600"
>
Confirm
</button>
</div>
</div>
</div>
{/if}
Using the Component
Here’s how to implement the modal component in a Svelte app:
<script>
import Modal from './Modal.svelte';
let showModal = false;
</script>
<button
class="bg-blue-500 text-white px-4 py-2 rounded"
on:click={() => showModal = true}
>
Open Modal
</button>
<Modal
isOpen={showModal}
title="Delete Item"
bind:isOpen={showModal}
>
<p>Are you sure you want to delete this item? This action cannot be undone.</p>
</Modal>
Performance Considerations
To ensure optimal performance:
- Tailwind: Automatically purges unused styles in production, keeping your CSS lightweight.
- Svelte: Compiles components into efficient JavaScript for fast load times.
- CSS Transitions: Use CSS animations for smoother modal interactions.
- Event Management: Properly clean up event listeners when components are destroyed.
Common Pitfalls to Avoid
When using Tailwind CSS and Svelte:
- Avoid Over-Nesting Classes: Keep class definitions concise for better readability.
- Ensure Accessibility: Implement proper keyboard navigation and ARIA attributes.
- Design Mobile-First: Start with mobile-friendly layouts to ensure responsive usability.